Unit 26 - Spatio-temporal LST scriptΒΆ
This unit is based on Unit 20 - MODIS LST and LST computation. The goal is to design a simple Python script for computing LST statistics in Norway area for given period. Input parameters:
- input - Name of the input space time raster dataset (line 6)
- start - Start date (line 9)
- end - End date (line 15)
- output - Name for output raster map with statistics (line 20)
A script outputs minimum, maximum and mean LST value. The raster map for computing statistics is created by t.rast.series (line 47) from input space time raster dataset. Statistics is computed by r.univar (line 57). Module output is formatted on lines 63-65.
In Unit 20 - MODIS LST space time dataset was created from data in 2017. It is reasonable also to check input dates range, see line 34.
Before running the script switch to mapset created Unit 20 - MODIS LST - modis. Procedure of changing current mapset is described in Workflow organization.
Tip
To decrease GRASS modules verbosity a quiet flag can be
set globally for the whole script by GRASS_VERBOSE
environment variable, see line 44. See GRASS
GIS documentation for other useful GRASS enviromental
variables.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | #!/usr/bin/env python
#%module
#% description: Computes LST stats for given period (limited to Germany and 2017).
#%end
#%option G_OPT_STRDS_INPUT
#%end
#%option
#% key: start
#% description: Start date 2017 (eg. 2017-03-01)
#% type: string
#% required: yes
#%end
#%option
#% key: end
#% description: End date 2017 (eg. 2017-04-01)
#% type: string
#% required: yes
#%end
#%option G_OPT_R_OUTPUT
#% description: Name for output raster map with statistics
#%end
import os
import sys
import atexit
from datetime import datetime
from subprocess import PIPE
import grass.script as gs
from grass.pygrass.modules import Module
from grass.exceptions import CalledModuleError
def check_date(date_str):
d = datetime.strptime(date_str, '%Y-%m-%d')
if d.year != 2017:
gs.fatal("Only year 2017 allowed")
def main():
check_date(options['start'])
check_date(options['end'])
# be silent
os.environ['GRASS_VERBOSE'] = '0'
try:
Module('t.rast.series',
input=options['input'],
output=options['output'],
method='average',
where="start_time > '{start}' and start_time < '{end}'".format(
start=options['start'], end=options['end']
))
except CalledModuleError:
gs.fatal('Unable to compute statistics')
ret = Module('r.univar',
flags='g',
map=options['output'],
stdout_=PIPE
)
stats = gs.parse_key_val(ret.outputs.stdout)
print('Min: {0:.1f}'.format(float(stats['min'])))
print('Max: {0:.1f}'.format(float(stats['max'])))
print('Mean: {0:.1f}'.format(float(stats['mean'])))
if __name__ == "__main__":
options, flags = gs.parser()
sys.exit(main())
|
Sample script to download: modis-date-stats.py
Example of usage:
modis-date-stats.py input=modis_c start=2017-03-01 end=2017-04-01 output=stats_sep
Min: -21.6
Max: 5.4
Mean: -9.4
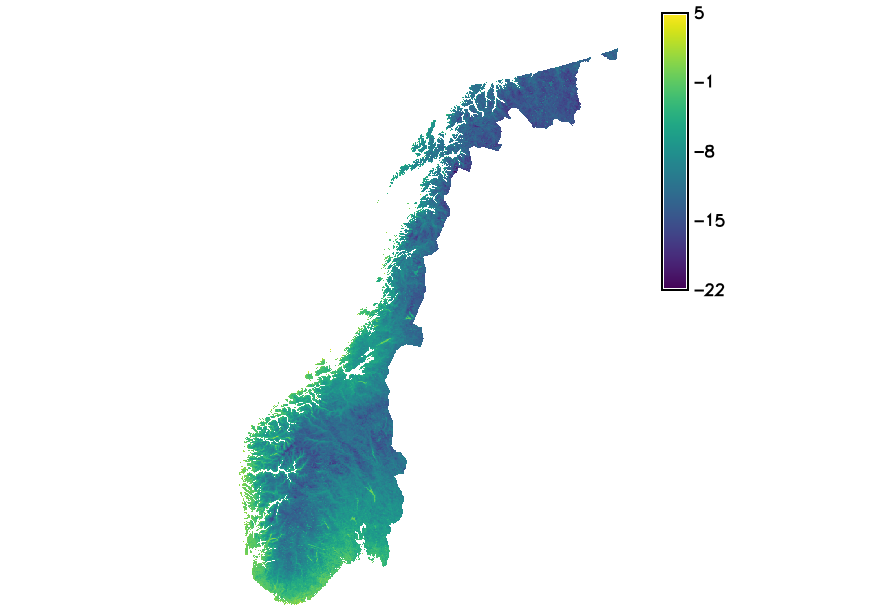
Fig. 123 Average LST in September produced by the script.